반응형
중복적인 코드를 제거하기 위해 파라미터 처리를 하여 테스트 코드를 수정.
@Test
public void testFree(){
// Given
Event event = Event.builder()
.basePrice(0)
.maxPrice(0)
.build();
// When
event.update();
// Then
assertThat(event.isFree()).isTrue();
// Given
event = Event.builder()
.basePrice(100)
.maxPrice(0)
.build();
// When
event.update();
// Then
assertThat(event.isFree()).isFalse();
// Given
event = Event.builder()
.basePrice(0)
.maxPrice(100)
.build();
// When
event.update();
// Then
assertThat(event.isFree()).isFalse();
}
2가지 방법으로 수정을 할 것이다.
우선 관련 어노테이션을 사용하기 위해 gradle기준 아래의 내용을 build.gradle에 넣어준다.
testImplementation group: 'pl.pragmatists', name: 'JUnitParams', version: '1.1.1'
참고 : https://github.com/Pragmatists/JUnitParams
maven repository : https://mvnrepository.com/artifact/pl.pragmatists/JUnitParams
@Test대신에 @ParameterizedTest로 대체한다. 그리고 @CsvSource로 basePrice, maxPrice, isFree 순서대로 값을 셋팅.
@ParameterizedTest
@CsvSource({
"0, 0, true",
"100,0, false",
"0, 100, false"
})
public void testFree(int basePrice, int maxPrice, boolean isFree){
// Given
event = Event.builder()
.basePrice(basePrice)
.maxPrice(maxPrice)
.build();
// When
event.update();
// Then
assertThat(event.isFree()).isEqualTo(isFree);
}
두번째 방법으로 타입이 명확하게 알수 있는 방법인데 아래와 같이 @MethodSource("메소드명")을 통해서 매개변수관련 메소드를
호출 한다.
@ParameterizedTest
@MethodSource("parametersFreeProvider")
public void testFree(int basePrice, int maxPrice, boolean isFree){
// Given
Event event = Event.builder()
.basePrice(basePrice)
.maxPrice(maxPrice)
.build();
// When
event.update();
// Then
assertThat(event.isFree()).isEqualTo(isFree);
}
static Stream<Arguments> parametersFreeProvider(){
return Stream.of(
arguments(0,0,true),
arguments(100,0,false),
arguments(0,100,false)
);
}
첫 소스에 비해 소스가 매우 깔끔해졌다.
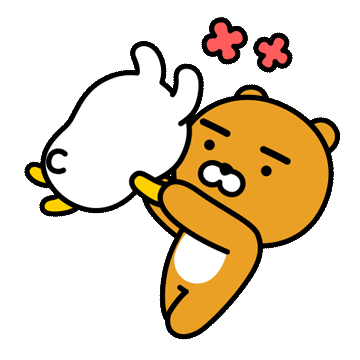
github : https://github.com/pthahaha/rest-api-study
반응형